Table of Contents
ToggleLet’s first explore what is lambda function in Python. The lambda function is anonymous. They are small functions without names. In Python normal functions start with the def keyword followed by name. However, the lambda function begins with the lambda keyword followed by the input parameters.
lambda [parameter_list]: expression
A parameter list is a list of parameters for lambda functions separated by a comma. Expression is a way to define a small anonymous function.
Why do we use the lambda function in Python? We use the lambda function to create short and throw-away functions as we need them briefly. They make the code more readable.
Lambda function with list comprehension in Python?
Using the lambda function with list comprehension shows the skills of a developer. We will first see the syntax of list comprehension.
[expression(variable) for variable in input_set [predicate][, …]]
- expression: Combination of values, variables, operations, and operations that evaluate to a single value.
- input_set: Represents input
- predicate: expression acting as a filter on members of the input set
If you want to know more about list comprehension follow here.
Now, that we know the syntax of both lambda function and list comprehension we can combine them. Let us start by taking a simple example. We want to square a list using lambda and list comprehension.
my_list = [1, 2, 3, 4, 5] squared_list = [(lambda x: x**2)(x) for x in my_list] print(squared_list)
Output:
[1, 4, 9, 16, 25]
We have declared a list. `lambda x: x**2` is the lambda function. The function has x as a parameter. The parameter is provided to the lambda function by iterating a list. In the above example, we replace the list comprehension expression part with a lambda function.
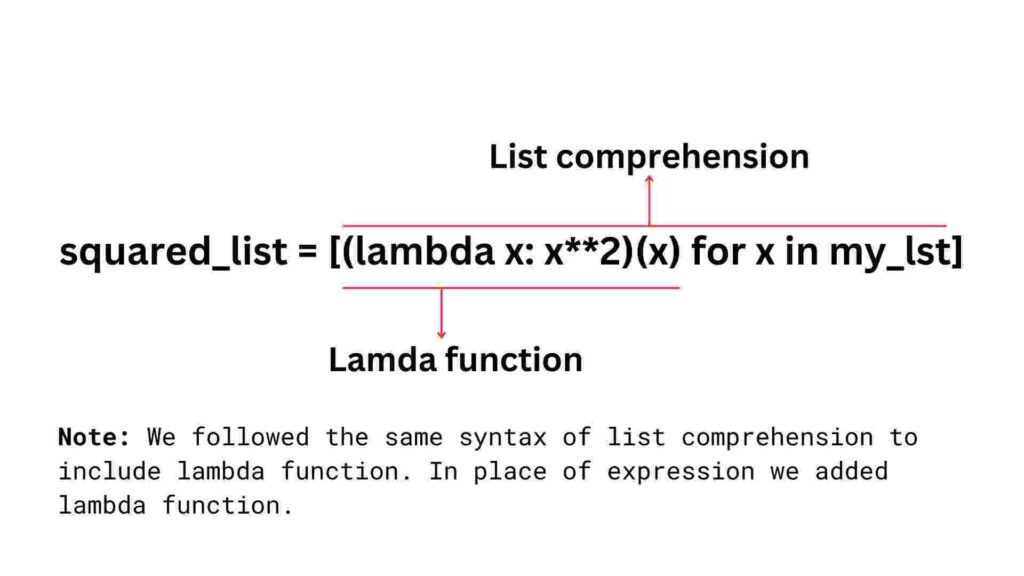
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_numbers = [x for x in my_list if (lambda x: x % 2 == 0)(x)] print(even_numbers)output
[2, 4, 6, 8, 10]Let us break down this code line ” [x for x in my_list if (lambda x: x % 2 == 0)(x)]”.
- We iterate through a list using this part of code “for x in my_list”.
- The lambda function “(lambda x: x % 2 == 0)” is used to check if x is an even number.
- The condition “if (lambda x: x % 2 == 0)(x)” filters out elements of the list by only keeping an even number. The whole statement creates a new list by keeping only even numbers
list1 = [1, 2, 3] list2 = [4, 5, 6] combined_list = [(lambda x, y: x + y)(x, y) for x, y in zip(list1, list2)] print(combined_list)Output:
[5, 7, 9]
Python lambda function with map function?
The map() function in Python is used to apply a given function to every item of an iterable like list, tuple, etc. We can also apply map function to the lambda function. Here is an example:
words = ["hello", "world", "python", "is", "awesome"] capitalized_words = map(lambda x: x.capitalize(), words) print(list(capitalized_words))
output
['Hello', 'World', 'Python', 'Is', 'Awesome']
In the above example, we capitalize words in the list. The map function takes two parameters: function and iterable.
We can combine these functions with list comprehension.
my_list = [1, 2, 3, 4, 5] squared_list = [x**2 for x in map(lambda x: x + 10, my_list)] print(squared_list)
output
[121, 144, 169, 196, 225]
We are mapping each element in my_list with a lambda function which adds 10 to the input parameter. After getting the value from the lambda function we square the value.
What is the scope of lambda function scope in Python?
The lambda function have their own local scope. They can access variables from the enclosing scope where they are defined. However, they cannot modify variables unless that variable is explicitly passed. Let us explore a example
g_v = 10 lambda_function = lambda x: x + g_v print(lambda_function(5))
Output
15
We have defined a lambda function that takes a single parameter x. It is also accessing a global variable g_v. It can only access that variable and it can not modify it.
FAQ
Can we use lambda in list comprehension?
Yes, we can use the lambda function in list comprehension. It makes your code more readable and concise. Let’s understand it with an example. We will reverse strings in the list.
string_list = ["apple", "banana", "cherry"] reversed_strings = [s[::-1] for s in map(lambda s: s , string_list)] print(reversed_strings)
Output:
['elppa', 'ananab', 'yrrehc']
- for s in string_list: iterates over the list containing a list of string.
- lambda s: s[::-1] : It is a lambda function which reverses a string
Combining both gives us a list with the word’s character in reverse order. This is how we can use the lambda function in list comprehension.
Is lambda faster than list comprehension Python?
The speed difference between using the lambda function and list comprehension is negligible in Python. Both the lambda and list comprehension are highly optimized constructs.
What is the benefit of using the lambda function in list comprehension?
- Lambda functions in Python are useful in a situation where you need a small anonymous function for a short time.
- Passing a function as an Argument: Lambda functions are handy when you need to pass a single function as an argument to another function. For example, we can pass the lambda function in the map and filter function.
- Making the code base more readable and concise.
When you should not use the lambda function in list comprehension?
- You should not use the lambda function in list comprehension in the following condition.
- If you are developing complex logic where you want to include multiple conditions and loops.
- These functions lack the name of the function making them less readable.
- Lambda functions are not meant for long-livin functions, and do not allow for modular extension. Ideally, it is recommended to create a Named Function to keep transformation login in one place, and can be re-used at multiple places which has better readability and maintainability of the code in our code base.
Conclusion
In this blog, we learned how to use the lambda function in list comprehension in Python. You can also see how to Solve object of type datetime is not JSON serializable in Python.
Pingback: Python Create a List of Size N with the Same Value -